Overview
In this lesson, you will learn to use Keypad. Keypad can be applied into various kinds of devices, including mobile phone, fax machine, microwave oven and so on. It is commonly used in user input.
Components Required
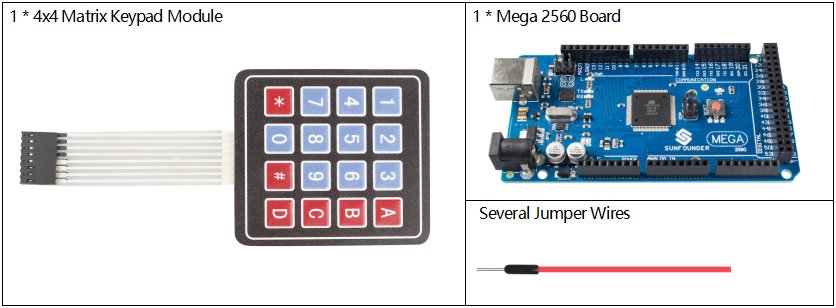
Component Introduction
A keypad is a rectangular array of 12 or 16 OFF-(ON) buttons. Their contacts are accessed via a header suitable for connection with a ribbon cable or insertion into a printed circuit board. In some keypads, each button connects with a separate contact in the header, while all the buttons share a common ground.
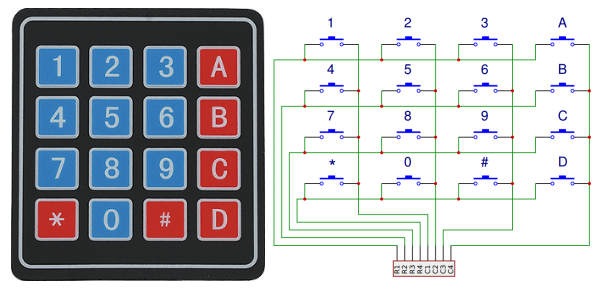
More often, the buttons are matrix encoded, meaning that each of them bridges a unique pair of conductors in a matrix. This configuration is suitable for polling by a microcontroller, which can be programmed to send an output pulse to each of the four horizontal wires in turn. During each pulse, it checks the remaining four vertical wires in sequence, to determine which one, if any, is carrying a signal. Pullup or pulldown resistors should be added to the input wires to prevent the inputs of the microcontroller from behaving unpredictably when no signal is present.
Fritzing Circuit
In this example, we extend the pins 1~8 of Keypad to connect to the digital pins 2~9.

Schematic Diagram
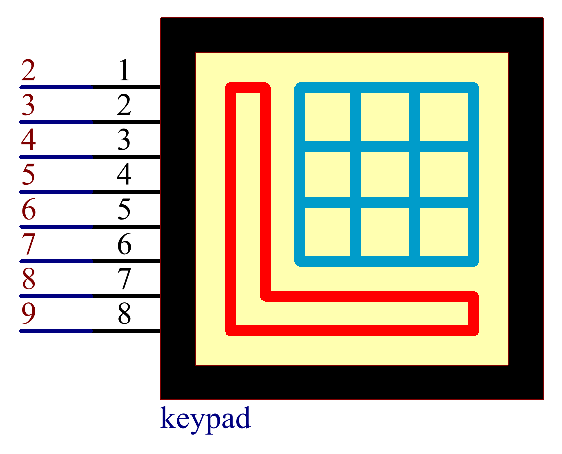
Code
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 4; //four columns
//define the cymbols on the buttons of the keypads
char hexaKeys[ROWS][COLS] =
{
{ '1','2','3','A' },
{ '4','5','6','B' },
{ '7','8','9','C' },
{ '*','0','#','D' }
};
byte rowPins[ROWS] = {2, 3, 4, 5}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {6, 7, 8, 9}; //connect to the column pinouts of the keypad
//initialize an instance of class NewKeypad
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
void setup(){
Serial.begin(9600);
}
void loop(){
char customKey = customKeypad.getKey();
if (customKey){
Serial.println(customKey);
}
}
The codes use the library Keypad.h. Please refer to Part 4 – 4.1 Add Libraries to import the library.
After uploading the codes to the Mega2560 board, on the serial monitor, you can see the value of the key currently pressed on the Keypad.
Code Analysis
By calling the Keypad.h library, you can easily use Keypad.
Library Functions:
Keypad(char *userKeymap, byte *row, byte *col, byte numRows, byte numCols)
Initializes the internal keymap to be equal to userKeymap.
userKeymap: The symbols on the buttons of the keypads.
row, col: Pin configuration.
numRows, numCols: Keypad sizes.
char getKey()
Returns the key that is pressed, if any. This function is non-blocking.
Phenomenon Picture
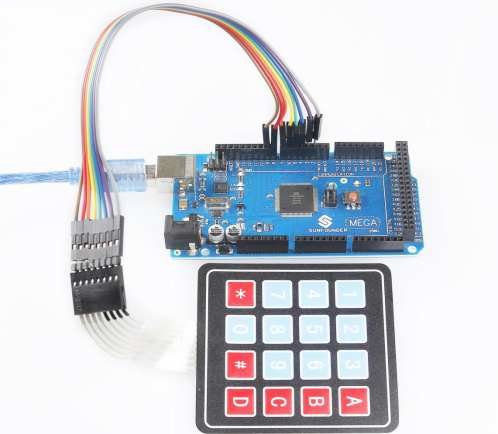