Overview
In this lesson, you will learn how to use MPR121. It’s a good option when you want to add a lot of touch switches to your project. The electrode of MPR121 can be extended with a conductor. If you connect a wire to a banana, you can turn the banana into a touch switch, thus realizing projects such as fruit piano.
Components Required
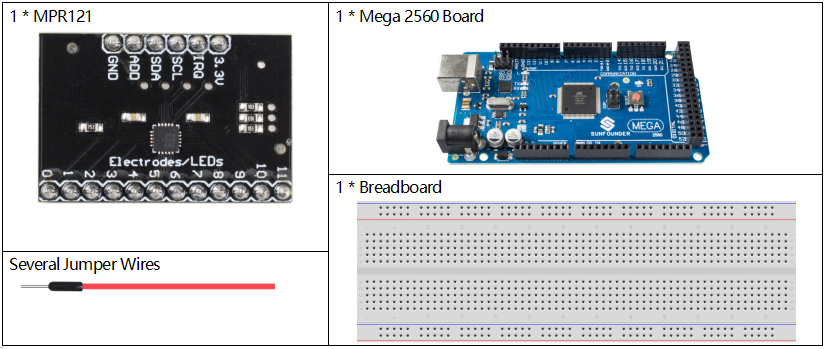
Component Introduction
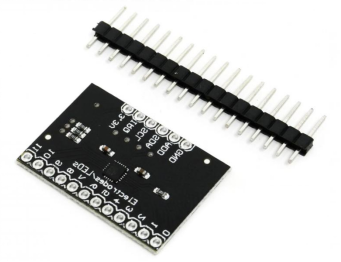
Add lots of touch sensors to your next project with this easy-to-use 12-channel capacitive touch sensor breakout board, starring the MPR121. This chip can handle up to 12 individual touch pads.
The MPR121 has support for only I2C, which can be implemented with nearly any microcontroller. You can select one of 4 addresses with the ADDR pin, for a total of 48 capacitive touch pads on one I2C 2-wire bus. Using this chip is a lot easier than doing the capacitive sensing with analog inputs: it handles all the filtering for you and can be configured for more/less sensitivity.
When the MPR121 senses a change, it pulls an interrupt pin LOW. The control board going to check that pin to see if it is LOW during the loop. To do this, this sensor also needs access to another digital pin.
Electrodes
Electrode is a touch sensor. Typically, electrodes can just be some piece of metal, or a wire. But some times depending on the length of our wire, or the material the electrode is on, it can make triggering the sensor difficult. For this reason, the MPR121 allows you to configure what is needed to trigger and untrigger an electrode.
Fritzing Circuit
In this example, we insert MPR121 into the breadboard. Get the GND of MPR121 connected to GND, 3.3V to 3V3, IRQ to the digital pin 2, SCL to the pin SCL(21), and SDA to the pin SDA(20). There are 12 electrodes for touch sensing. Note: MPR121 is powered by 3.3V, not 5V.
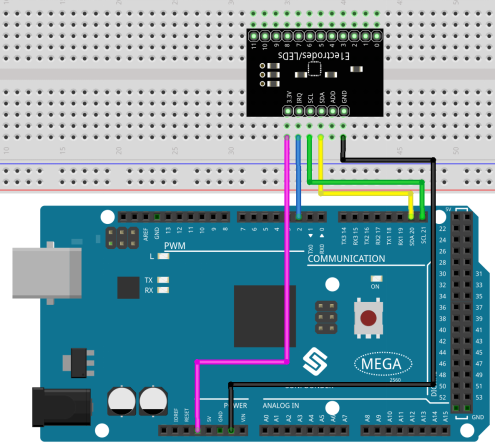
Schematic Diagram
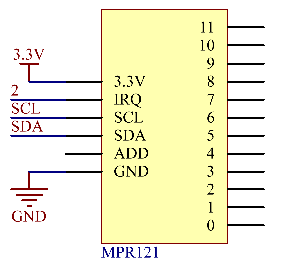
Code
The codes use the MPR121_JM. h library. Turn to Part 4 – 4.1 Add Libraries for information on how to import Libraries.
#include <MPR121_JM.h>
MPR121 mpr121(2, 0x2A, 0x1F);
boolean touchStates[12];
void setup() {
mpr121.mpr121_setup();
Serial.begin(9600);
}
void loop() {
if (!mpr121.checkInterrupt())
{
int touched = mpr121.readTouchInputs();
for (int i = 0; i < 12; i++) {
if (touched & (1 << i)) {touchStates[i] = 1;}
else {touchStates[i] = 0;}
}
for (int i = 0; i < 12; i++)
{Serial.print(touchStates[i]);}
Serial.println();
}
}
After uploading the codes to the Mega2560 board, the touch state of pins of MPR121 「1」and「0」will be recorded in a 12 – bit boolean type of array that will be printed on the serial monitor.
Code Analysis
The function of the module is included in the library MPR121_JM.h.
#include <MPR121_JM.h>
Library Functions:
MPR121(int irqpin,uint8_t touThresh,uint8_t relThresh)
Creates a new instance of the MPR121.
irqpin: the interrupt request pin.
touThresh: Touch threshold,the electrode is recognized as a threshold of the 「Touch」 state.
relThresh: Release threshold,the electrode is recognized as a threshold of the 「Release」 state.
The range of the electrode data value is 0~255. For typical touch application, the threshold value can be in range 0x05~0x30 for example. The smaller the value, the more sensitive it is. The setting of the threshold is depended on the actual application.Typically the touch threshold is a little bigger than the release threshold to touch debounce and hysteresis.
void mpr121_setup()
Setup MPR121 module.
bool checkInterrupt()
Make interrupt judgment, when the electrode state changes, the function returns a Boolean value 「0」.
uint16_t readTouchInputs()
The touch state of the electrode produces a Boolean value. The function accumulates the Boolean values generated by all the electrodes in sequence into a 12-bit binary number as the return value. If the first and eleventh electrodes are touched, 「100000000010」 is returned.
Phenomenon Picture
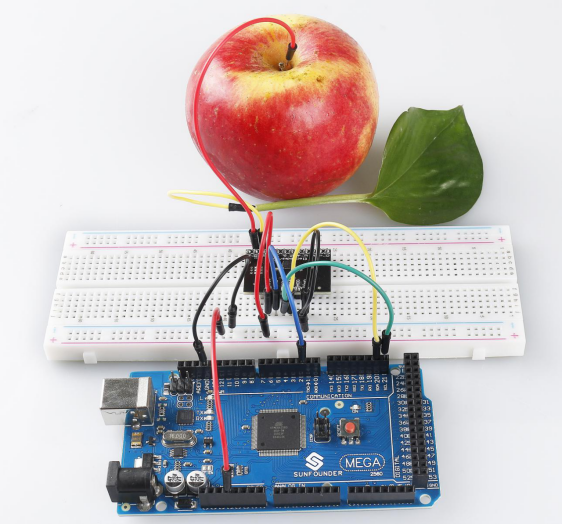