Introduction
In this lesson, we will learn how to use buttons.
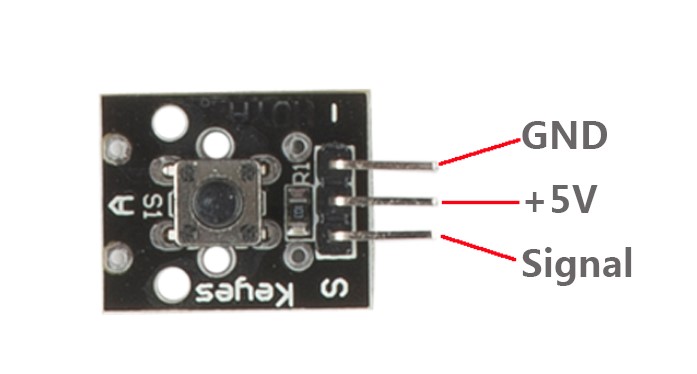
Components
– 1 * Raspberry Pi
– 1 * Breadboard
– 1 * Network cable (or USB wireless network adapter)
– 1 * Button module
– 1 * Dual-color Common-Cathode LED module
– Several jumper wires
Experimental Principle
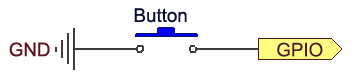
Use a normally open button as an input device of Raspberry Pi. When the button is pressed, the General Purpose Input/Output (GPIO) connected to the button will turn into low level (0V). We can detect the state of the GPIO connected to the button through programming. That is, if the GPIO turns into low level, it means the button is pressed. Then you can run the corresponding code accordingly.
In this experiment, we will print a string on the screen and control an LED.
Experimental Procedures
Step 1: Build the circuit
Button Module Raspberry Pi
S ——————————– GPIO0
+ ——————————- 3.3V
– ——————————– GND
Dual-color LED module connection: connect pin R on dual-color LED module to GPIO1 of the Raspberry Pi; GND to GND
Step 2: Edit and save the code (see path/Rpi_SensorKit_code/13_button/button.c)
Step 3: Compile
gcc button.c -lwiringPi
Step 4: Run
./a.out
Press the button and you will see a string “Button is pressed” displayed on the screen, and the state of the LED will be switched ON/OFF.
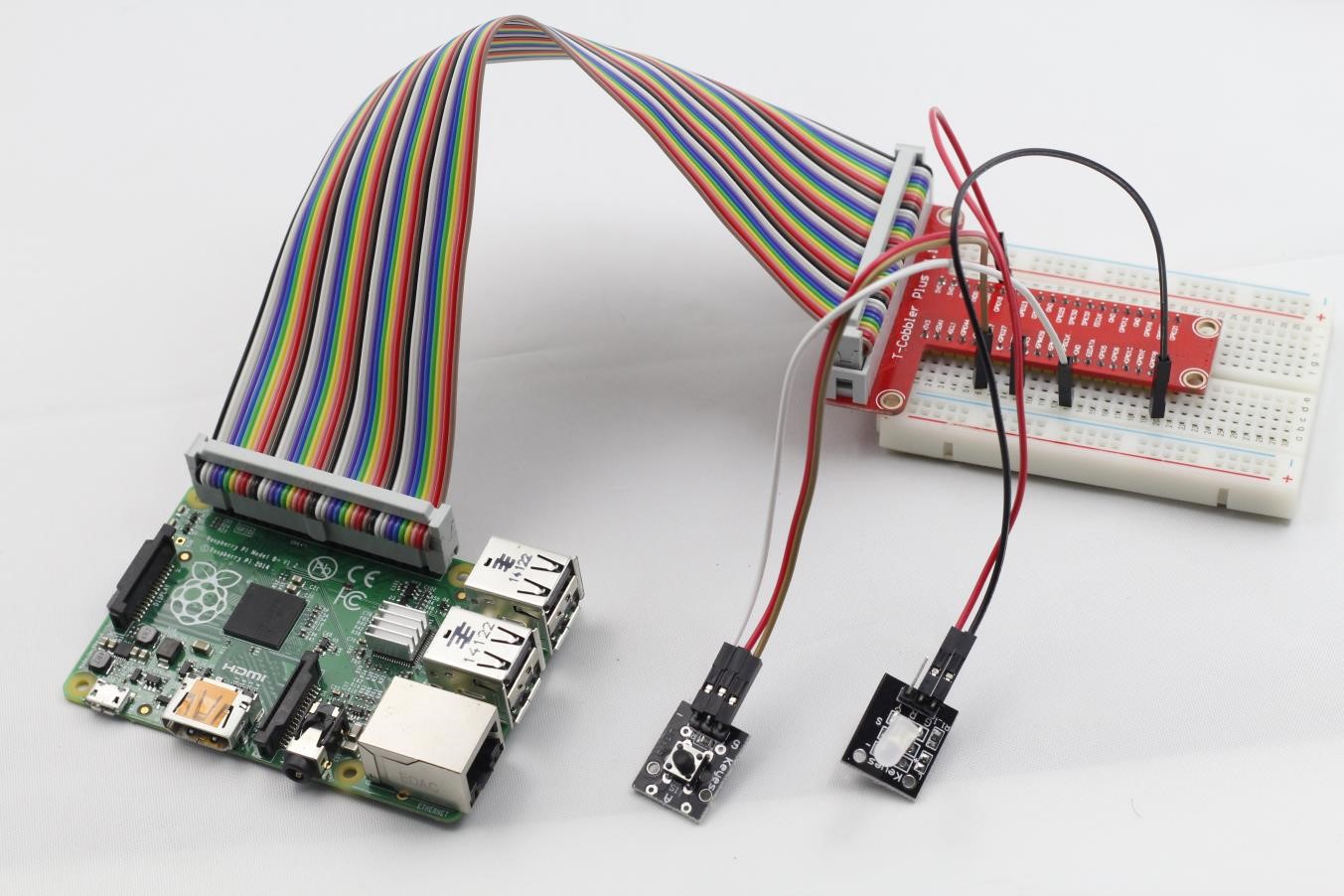
button.c
#include <wiringPi.h>
#include <stdio.h>
#define BtnPin 0
#define LedPin 1
int main(void)
{
if(wiringPiSetup() == -1){ //when initialize wiring failed,print messageto screen
printf("setup wiringPi failed !");
return 1;
}
pinMode(BtnPin, INPUT);
pinMode(LedPin, OUTPUT);
while(1){
if(0 == digitalRead(BtnPin)){
delay(10);
if(0 == digitalRead(BtnPin)){
while(!digitalRead(BtnPin));
digitalWrite(LedPin, !digitalRead(LedPin));
printf("Button is pressed\n");
}
}
}
return 0;
}
Python Code
#!/usr/bin/env python
import RPi.GPIO as GPIO
BtnPin = 11
LedPin = 12
Led_status = 0
def setup():
GPIO.setmode(GPIO.BOARD) # Numbers GPIOs by physical location
GPIO.setup(LedPin, GPIO.OUT) # Set LedPin's mode is output
GPIO.setup(BtnPin, GPIO.IN, pull_up_down=GPIO.PUD_UP) # Set BtnPin's mode is input, and pull up to high level(3.3V)
GPIO.output(LedPin, GPIO.LOW) # Set LedPin low to off led
def swLed(ev=None):
global Led_status
Led_status = not Led_status
GPIO.output(LedPin, Led_status) # switch led status(on-->off; off-->on)
print "LED: on " if Led_status else "LED: off"
def loop():
GPIO.add_event_detect(BtnPin, GPIO.FALLING, callback=swLed, bouncetime=200) # wait for falling
while True:
pass # Don't do anything
def destroy():
GPIO.output(LedPin, GPIO.LOW) # led off
GPIO.cleanup() # Release resource
if __name__ == '__main__': # Program start from here
setup()
try:
loop()
except KeyboardInterrupt: # When 'Ctrl+C' is pressed, the child program destroy() will be executed.
destroy()