Introduction
There are two same Magic Cup modules in this kit, and each adds a separate LED based on the mercury switch. You may learn the application of one module and then try to apply two modules together to make one dim when at the same time the other brightens.
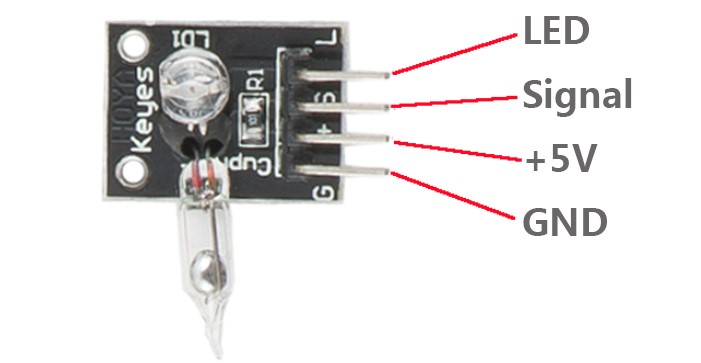
Components
– 1 * Raspberry Pi
– 1 * Breadboard
– 1 * Network cable (or USB wireless network adapter)
– 2 * Magic cup module
– Several jumper wires
Experimental Principle
Tilt the module and the LED onside will dim gradually. The working principle is similar to that of the Mercury Switch.
Experimental Procedures
Step 1: Build the circuit
Magic Cup Raspberry Pi
L ———————————— GPIO1
S ———————————— GPIO0
+ ———————————— 3.3V
G ———————————— GND
Step 2: Edit and save the code (see path/Rpi_SensorKit_code/29_magicCup/magic.c)
Step 3: Compile
gcc magic.c -lwiringPi
Step 4: Run
./a.out
Now, tilt the magic cup and the LED on one module will get dim.
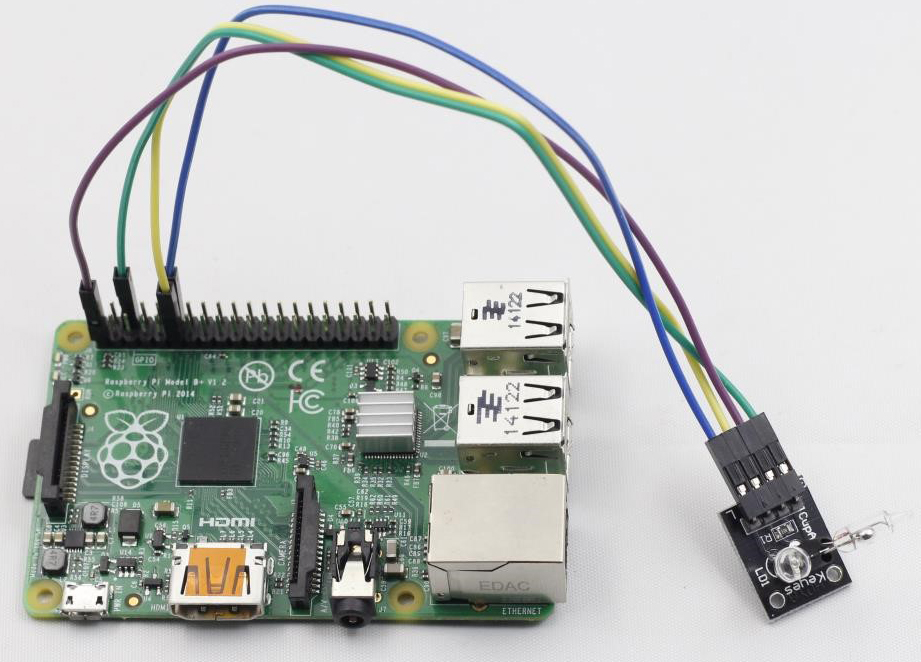
magic.c
#include <wiringPi.h>
#include <stdio.h>
#define SIG 0
#define LED 1
int main(void)
{
if(wiringPiSetup() == -1){
printf("setup wiringPi failed !");
return 1;
}
pinMode(SIG, INPUT);
pinMode(LED, OUTPUT);
while(1){
if(0 == digitalRead(SIG)){
digitalWrite(LED, 1);
}
else{
digitalWrite(LED, 0);
}
}
return 0;
}
Python Code
#!/usr/bin/env python
import RPi.GPIO as GPIO
import time
MERCURY_SIG1 = 11
LED1 = 12
LED2 = 15
def setup():
global p_LED1, p_LED2
GPIO.setmode(GPIO.BOARD)
GPIO.setup(MERCURY_SIG1, GPIO.IN)
GPIO.setup(LED1, GPIO.OUT)
GPIO.setup(LED2, GPIO.OUT)
p_LED1 = GPIO.PWM(LED1, 200)
p_LED2 = GPIO.PWM(LED2, 200)
p_LED1.start(0)
p_LED2.start(0)
def loop():
led1_value = 0
led2_value = 0
while True:
mercury_value = GPIO.input(MERCURY_SIG1)
if mercury_value == 0:
led1_value -= 1
led2_value += 1
if led1_value < 0:
led1_value = 0
if led2_value > 100:
led2_value = 100
if mercury_value == 1:
led1_value += 1
led2_value -= 1
if led1_value > 100:
led1_value = 100
if led2_value < 0:
led2_value = 0
p_LED1.ChangeDutyCycle(led1_value)
p_LED2.ChangeDutyCycle(led2_value)
time.sleep(0.05)
def destroy():
p_LED1.stop()
p_LED2.stop()
GPIO.cleanup()
if __name__ == "__main__":
try:
setup()
loop()
except KeyboardInterrupt:
destroy()