Introduction
A potentiometer is a device which is used to vary the resistance in an electrical circuit without interrupting the circuit.
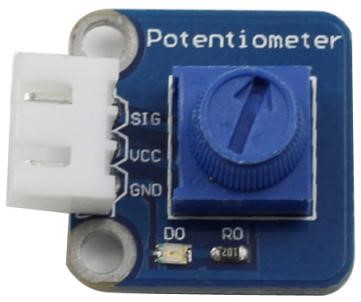
Components
– 1 * Raspberry Pi
– 1 * Breadboard
– 1 * Network cable (or USB wireless network adapter)
– 1 * Potentiometer module
– 1 * Dual-Color LED module
– 2 * 3-Pin anti-reverse cable
– Several Jumper wires (M to F)
Experimental Principle
An analog potentiometer is an analog electronic component. What’s the difference between an analog one and a digital one? Simply put, a digital potentiometer refers to just two states like on/off, high/low levels, i.e. either 0 or 1, while a digital one supports analog signals like a number from 1 to 1000. The signal value changes over time instead of keeping an exact number. Analog signals include light intensity, humidity, temperature, and so on.
The schematic diagram:
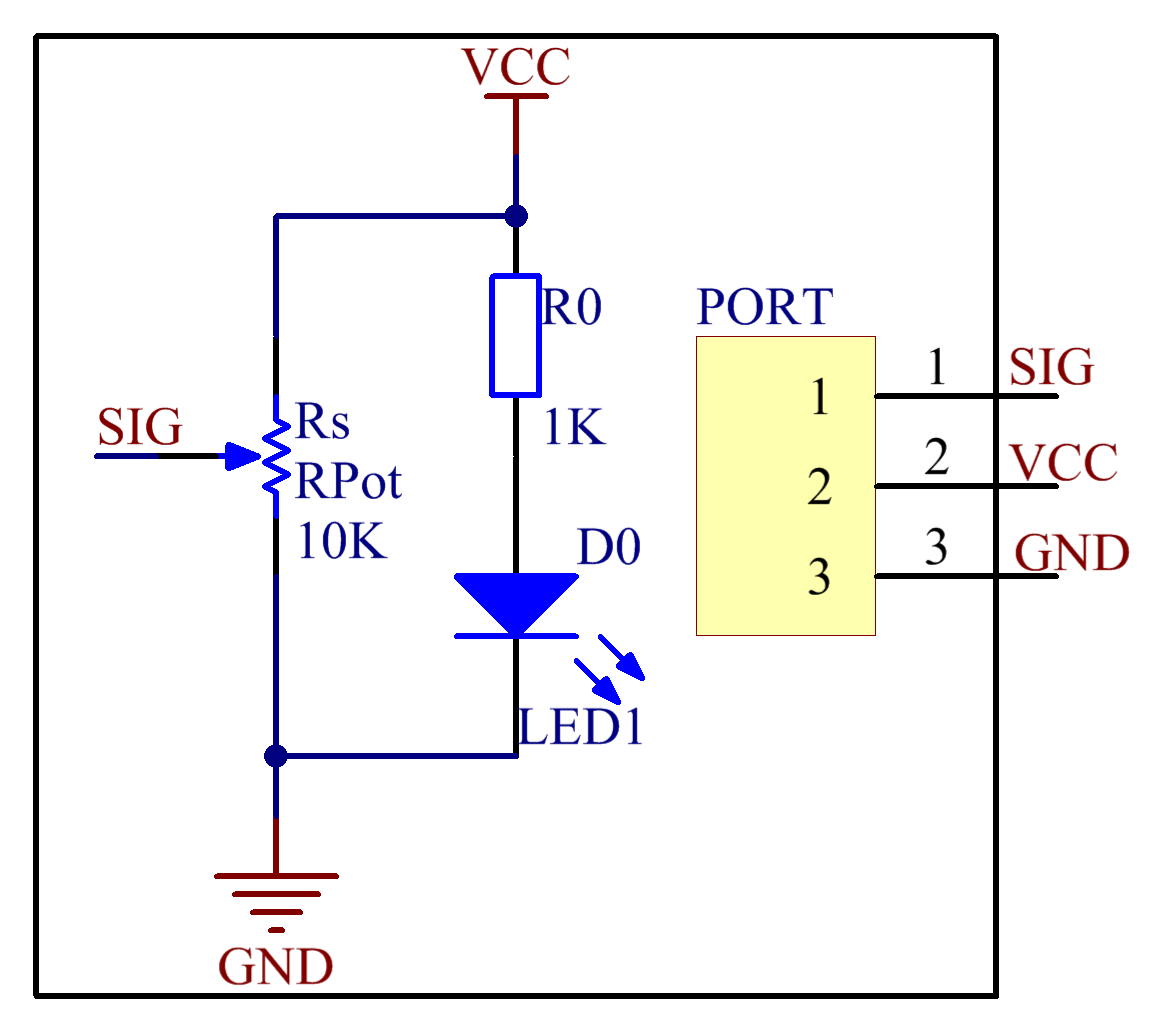
Experimental Procedures
Step 1: Build the circuit
Raspberry Pi | PCF8591 Module | Potentiometer |
SDA | SDA | * |
SCL | SCL | * |
3V3 | VCC | VCC |
GND | GND | GND |
* | AIN0 | SIG |
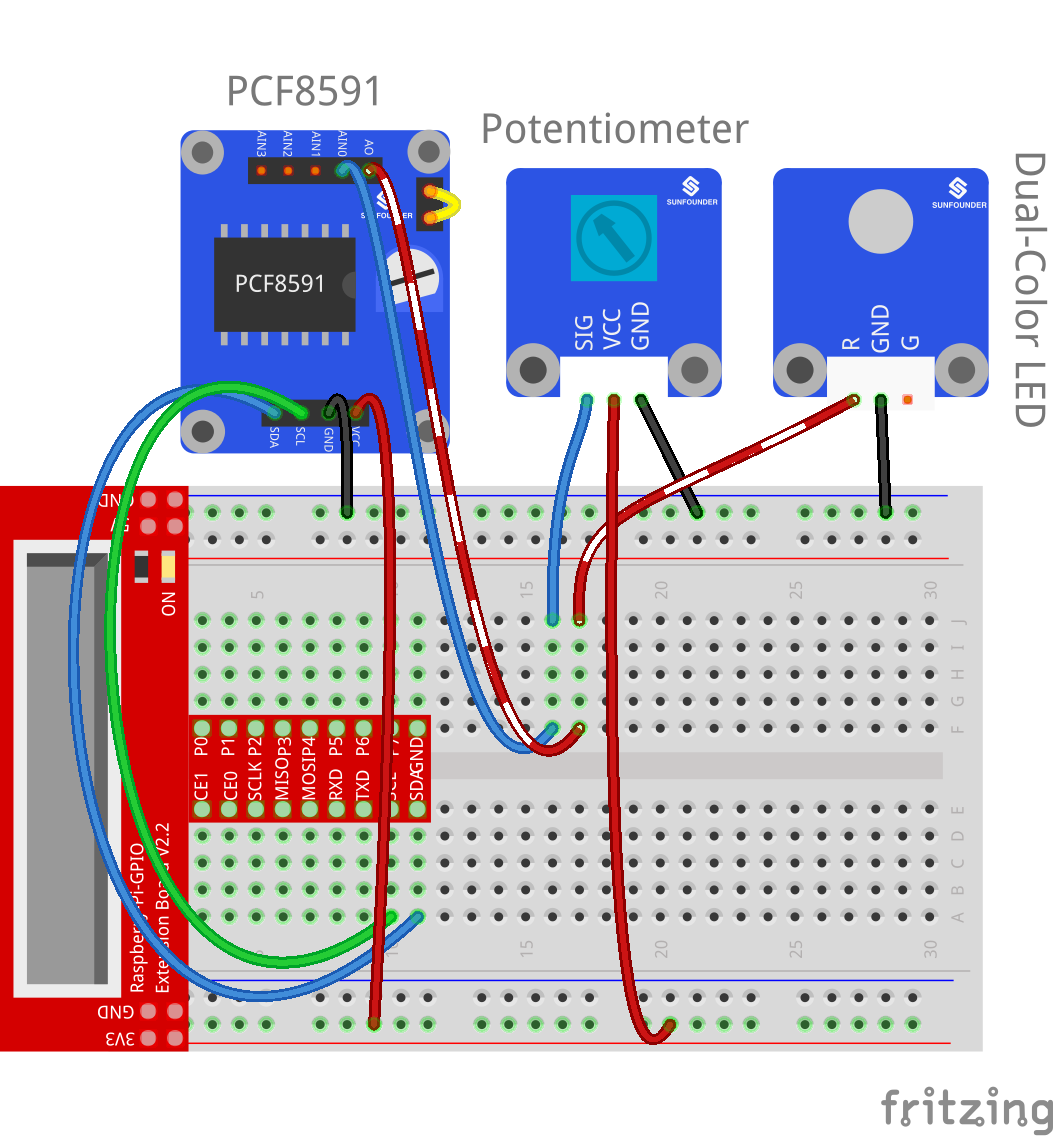
For C language users:
Step 2: Change directory
cd /home/pi/SunFounder_SensorKit_for_RPi2/C/16_potentiometer/
Step 3: Compile
gcc potentiometer.c -lwiringPi
Step 4: Run
sudo ./a.out
For Python users:
Step 2: Change directory
cd /home/pi/SunFounder_SensorKit_for_RPi2/Python/
Step 3: Run
sudo python 16_potentiometer.py
Turn the shaft of the potentiometer, and you can see the value printed on the screen change from 0 (minimum) to 255 (maximum).
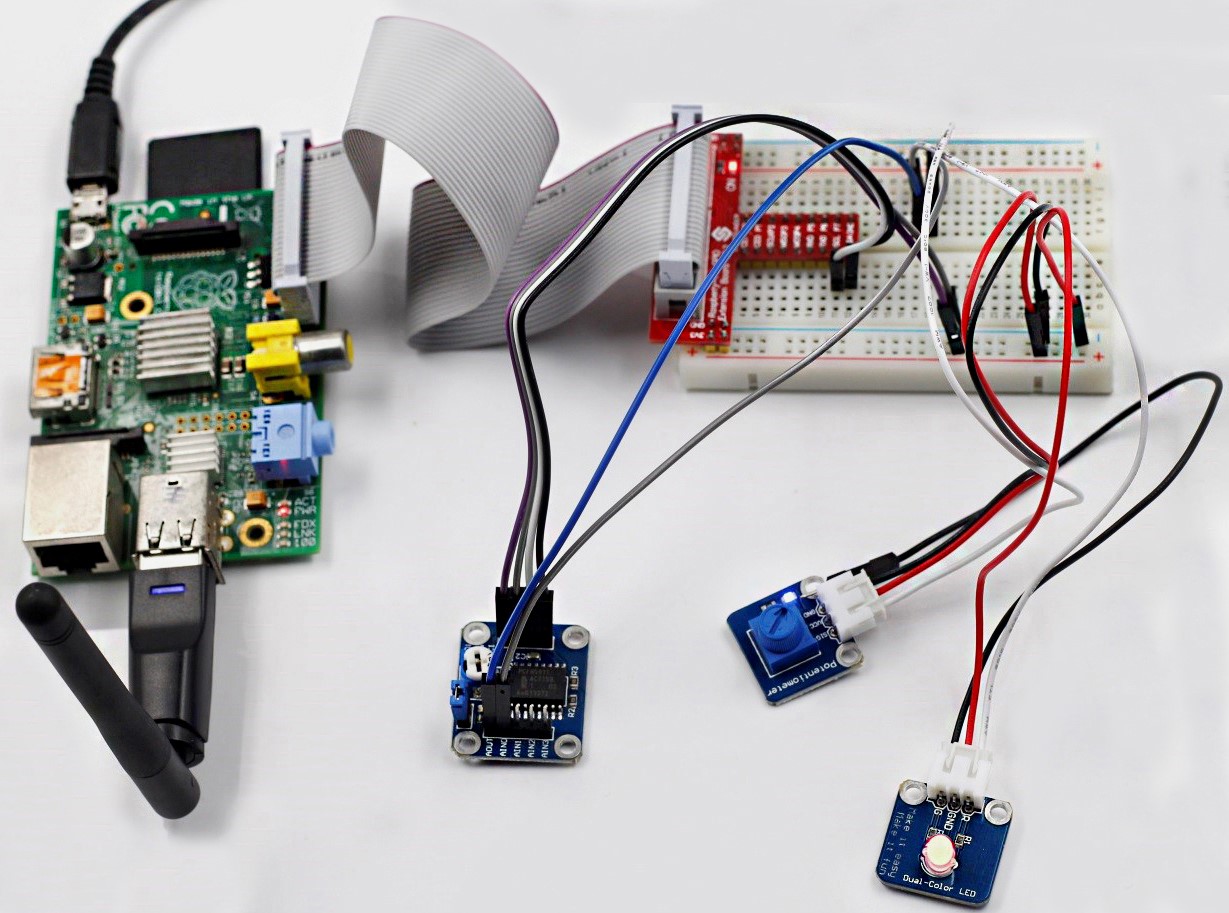
C Code
#include <stdio.h>
#include <wiringPi.h>
#include <pcf8591.h>
#define PCF 120
int main (void)
{
int value ;
wiringPiSetup () ;
// Setup pcf8591 on base pin 120, and address 0x48
pcf8591Setup (PCF, 0x48) ;
while(1) // loop forever
{
value = analogRead (PCF + 0) ;
printf("Value: %d\n", value);
analogWrite (PCF + 0, value) ;
delay (200) ;
}
return 0 ;
}
Python Code
#!/usr/bin/env python
import PCF8591 as ADC
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
def setup():
ADC.setup(0x48)
def loop():
status = 1
while True:
print 'Value:', ADC.read(0)
time.sleep(0.2)
if __name__ == '__main__':
try:
setup()
loop()
except KeyboardInterrupt:
pass