Introduction
A flame sensor module performs detection by capturing infrared wavelengths from flame. It can be used to detect and warn of flames.
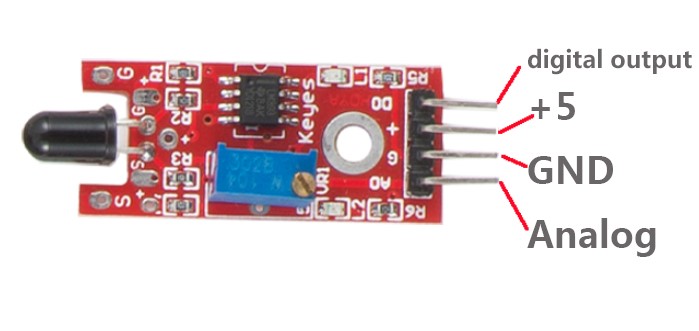
Components
– 1 * Raspberry Pi
– 1 * Network cable (or USB wireless network adapter)
– 1 * Flame sensor module
– Several jumper wires
Experimental Principle
There are several types of flame sensors. In this experiment, we will use a far-infrared flame sensor. It can detect infrared light with a wavelength ranging from 700nm to 1000nm. A far-infrared flame probe converts the strength changes of the external infrared light into current changes. And then it converts analog quantities into digital ones.
In this experiment, connect pin DO of flame sensor module to a GPIO of Raspberry Pi to detect flame existence by programming.
Experimental Procedures
Step 1: Build the circuit
Raspberry Pi Flame Sensor
GPIO0 ——————————– DO
3.3V ——————————— +
GND ——————————— G
Step 2: Edit and save the code (see path/Rpi_SensorKit_code/25_flameSensor/flameSensor.c)
Step 3: Compile
gcc flameSensor.c -lwiringPi
Step 4: Run
./a.out
Now, ignite a lighter in the range of 80 cm near the module and a string “Detected Flame!” will be displayed on the screen.
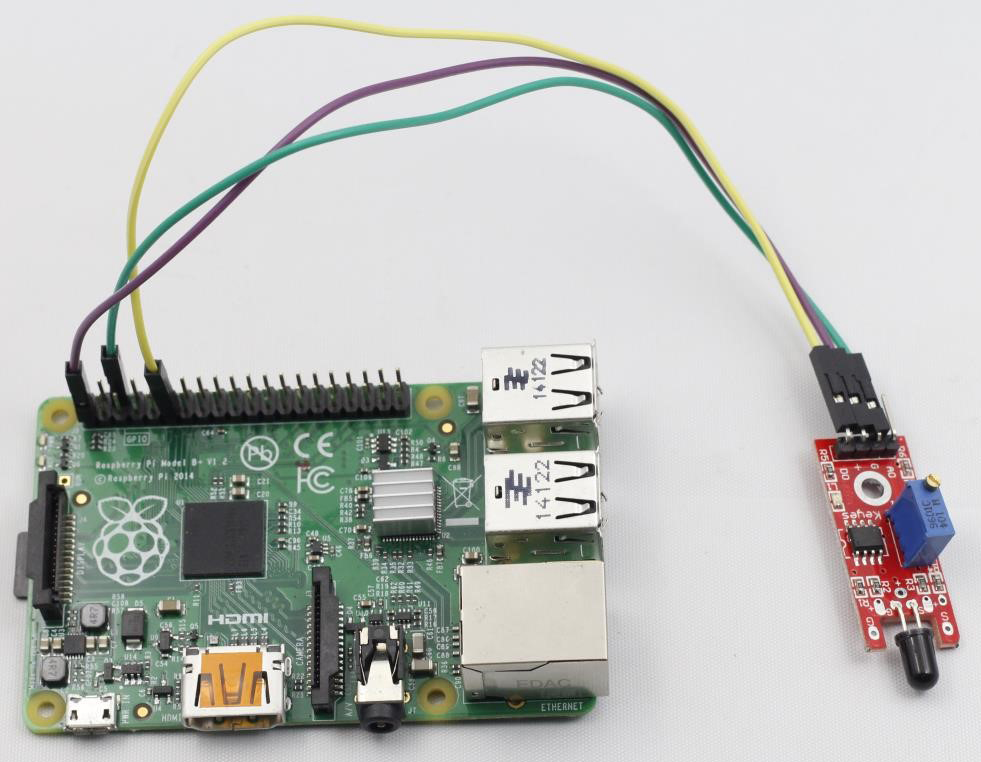
flameSensor.c
#include <wiringPi.h>
#include <stdio.h>
#define FlamePin 0
void myISR(void)
{
printf("Detected Flame !\n");
}
int main(void)
{
if(wiringPiSetup() == -1){ //when initialize wiring failed,print messageto screen
printf("setup wiringPi failed !\n");
return 1;
}
if(wiringPiISR(FlamePin, INT_EDGE_FALLING, &myISR)){
printf("setup interrupt failed !\n");
return 1;
}
while(1){
;
}
return 0;
}
Python Code
#!/usr/bin/env python
import RPi.GPIO as GPIO
import time
FlamePin = 11
def init():
GPIO.setmode(GPIO.BOARD)
GPIO.setup(FlamePin, GPIO.IN, pull_up_down=GPIO.PUD_UP)
def myISR(ev=None):
print "Flame is detected !"
def loop():
GPIO.add_event_detect(FlamePin, GPIO.FALLING, callback=myISR)
while True:
pass
if __name__ == '__main__':
init()
try:
loop()
except KeyboardInterrupt:
print 'The end !'