Introduction
In this lesson, we will learn how to make eight LEDs blink in various effects as you want based on Raspberry Pi.
Components
– 1 * Raspberry Pi
– 1 * Breadboard
– 8 * LED
– 8 * Resistor (220Ω)
– Jumper wires
– 1 * T-Extension Board
– 1 * 40-Pin Cable
Principle
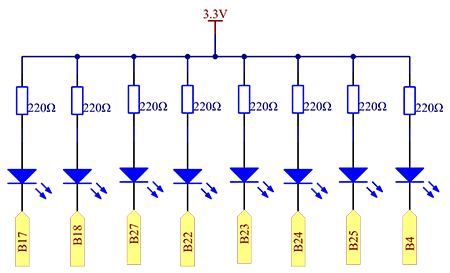
Principle: Judging from the schematic diagram, we can know that a LED and a current-limiting resistor have been connected to B17, B18, B27, B22, B23, B24, B25, and B4 respectively. The current-limiting resistor has been connected to the 3.3V power supply on other side. Therefore, if we want to light up one LED, we only need to set the GPIO of the LED as low level. So in this experiment, set B17, B18, B27, B22, B23, B24, B25, and B4 to low level in turn by programming, and then LED0-LED7 will light up in turn. You can make eight LEDs blink in different effects by controlling their delay time and the order of lighting up.
Experimental Procedures
Step 1: Build the circuit
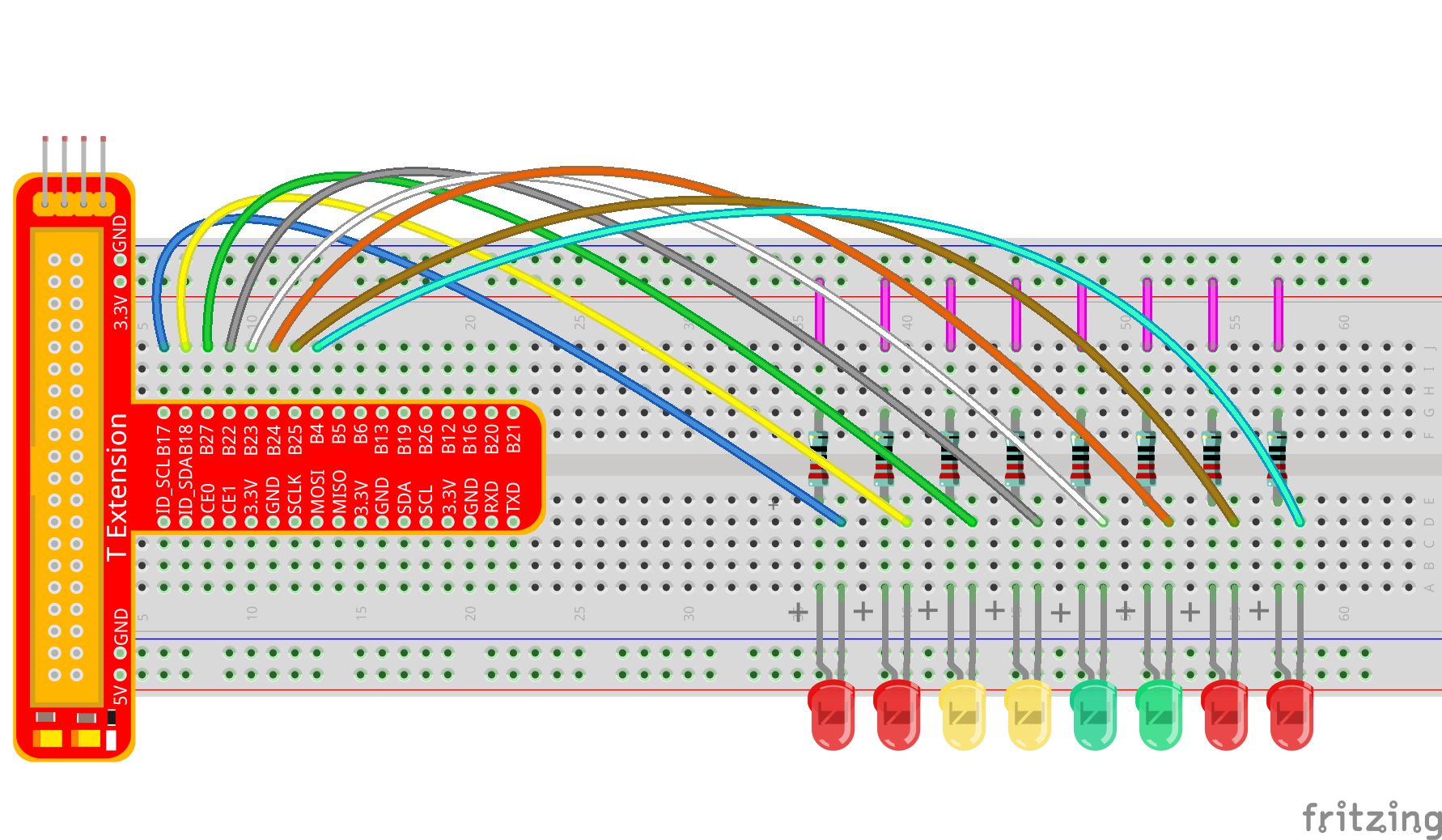
For C language users:
Step 2: Open the code file
cd /home/pi/SunFounder_Super_Kit_V3.0_for_Raspberry_Pi/C
Note: Use the cd command to switch to the code path of this experiment.
Step 3: Compile the Code
gcc 03_8Led.c -o 03_8Led -lwiringPi
or
make 03_8Led
Note: gcc is a linux command which can realize compiling and generating the C language program file 03_8Led.c to the executable file 03_8Led.
make is a linux command which can compiling and generating the executable file according to the rule inside the makefile.
Step 4: Run the executable file above
sudo ./03_8Led
Note: Here the Raspberry Pi will run the executable file 03_8Led compiled previously.
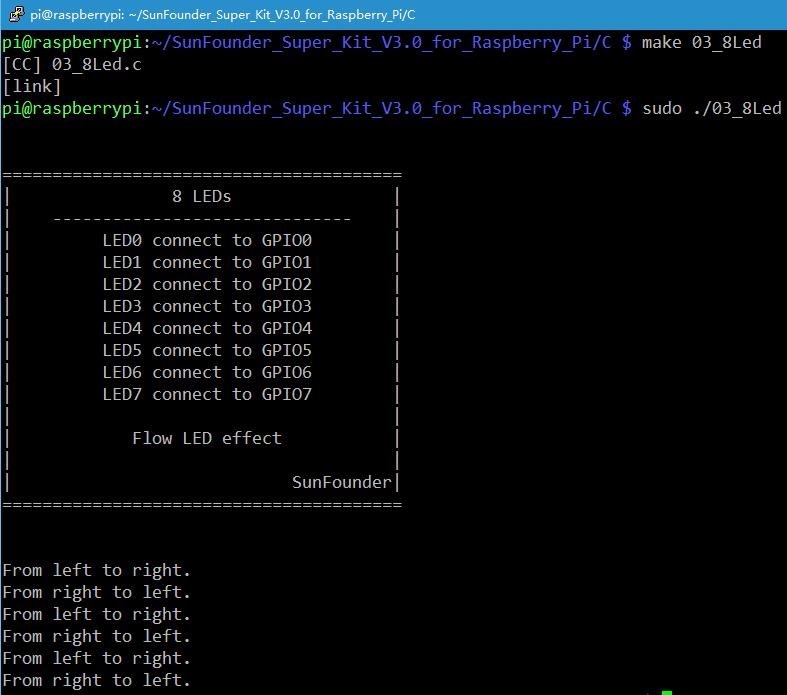
For Python users:
Step 2: Open the code file
cd /home/pi/SunFounder_Super_Kit_V3.0_for_Raspberry_Pi/Python
Step 3: Run
sudo python 03_8Led.py
You will see the eight LEDs lighten up one by one, and then dim in turn.
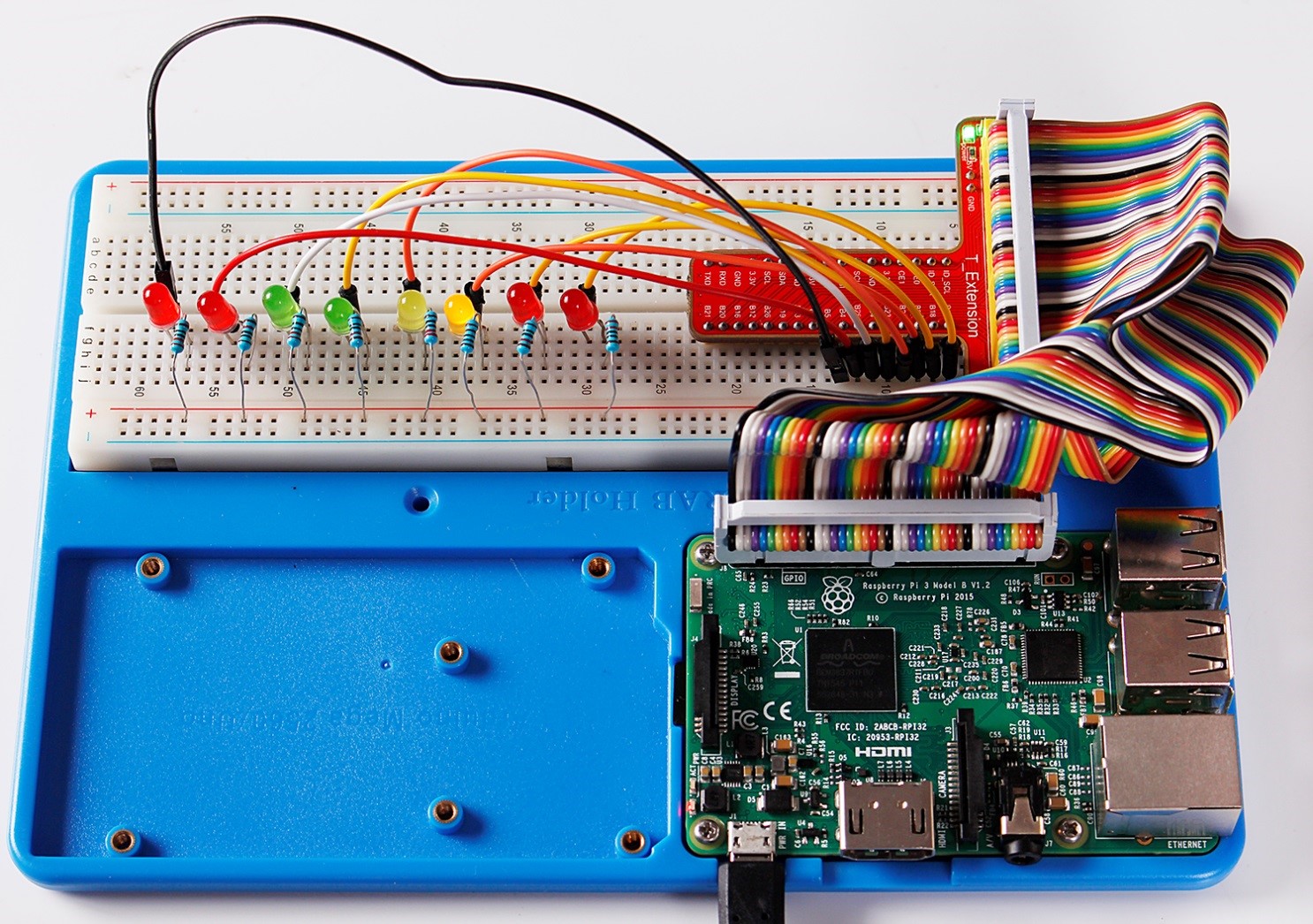
Further Exploration
You can write the blinking effects of LEDs in an array. If you want to use one of these effects, you can call it in the main() function directly.
C Code
/**********************************************************************
* Filename : 8Led.c
* Description : Make a breathing led.
* Author : Robot
* E-mail : support@sunfounder.com
* website : www.sunfounder.com
* Update : Cavon 2016/07/01
**********************************************************************/
#include <wiringPi.h>
#include <stdio.h>
// Turn LED(channel) on
void led_on(int channel){
digitalWrite(channel, LOW);
}
// Turn LED(channel) off
void led_off(int channel){
digitalWrite(channel, HIGH);
}
int main(void){
int i;
// When initialize wiring failed, print messageto screen
if(wiringPiSetup() == -1){
printf("setup wiringPi failed !");
return 1;
}
// Set 8 pins' modes to output
for(i=0;i<8;i++){
pinMode(i, OUTPUT);
}
printf("\n");
printf("\n");
printf("========================================\n");
printf("| 8 LEDs |\n");
printf("| ------------------------------ |\n");
printf("| LED0 connect to GPIO0 |\n");
printf("| LED1 connect to GPIO1 |\n");
printf("| LED2 connect to GPIO2 |\n");
printf("| LED3 connect to GPIO3 |\n");
printf("| LED4 connect to GPIO4 |\n");
printf("| LED5 connect to GPIO5 |\n");
printf("| LED6 connect to GPIO6 |\n");
printf("| LED7 connect to GPIO7 |\n");
printf("| |\n");
printf("| Flow LED effect |\n");
printf("| |\n");
printf("| SunFounder|\n");
printf("========================================\n");
printf("\n");
printf("\n");
while(1){
// Turn LED on from left to right
printf("From left to right.\n");
for(i=0;i<8;i++){
led_on(i);
delay(100);
led_off(i);
}
// Turn LED off from right to left
printf("From right to left.\n");
for(i=8;i>=0;i--){
led_on(i);
delay(100);
led_off(i);
}
}
return 0;
}
Python Code
#!/usr/bin/env python
import RPi.GPIO as GPIO
import time
# Set 8 Pins for 8 LEDs.
LedPins = [17, 18, 27, 22, 23, 24, 25, 4]
# Define a function to print message at the beginning
def print_message():
print ("========================================")
print ("| 8 LEDs |")
print ("| ------------------------------ |")
print ("| LED0 connect to GPIO0 |")
print ("| LED1 connect to GPIO1 |")
print ("| LED2 connect to GPIO2 |")
print ("| LED3 connect to GPIO3 |")
print ("| LED4 connect to GPIO4 |")
print ("| LED5 connect to GPIO5 |")
print ("| LED6 connect to GPIO6 |")
print ("| LED7 connect to GPIO7 |")
print ("| |")
print ("| Flow LED effect |")
print ("| |")
print ("| SunFounder|")
print ("========================================\n")
print 'Program is running...'
print 'Please press Ctrl+C to end the program...'
raw_input ("Press Enter to begin\n")
# Define a setup function for some setup
def setup():
# Set the GPIO modes to BCM Numbering
GPIO.setmode(GPIO.BCM)
# Set all LedPin's mode to output,
# and initial level to High(3.3v)
GPIO.setup(LedPins, GPIO.OUT, initial=GPIO.HIGH)
# Define a main function for main process
def main():
# Print messages
print_message()
leds = ['-', '-', '-', '-', '-', '-', '-', '-']
while True:
# Turn LED on from left to right
print "From left to right."
for pin in LedPins:
#print pin
GPIO.output(pin, GPIO.LOW)
leds[LedPins.index(pin)] = 0 # Show which led is on
print leds
time.sleep(0.1)
GPIO.output(pin, GPIO.HIGH)
leds[LedPins.index(pin)] = '-' # Show the led is off
# Turn LED off from right to left
print "From right to left."
for pin in reversed(LedPins):
#print pin
GPIO.output(pin, GPIO.LOW)
leds[LedPins.index(pin)] = 0 # Show which led is on
print leds
time.sleep(0.1)
GPIO.output(pin, GPIO.HIGH)
leds[LedPins.index(pin)] = '-' # Show the led is off
# Define a destroy function for clean up everything after
# the script finished
def destroy():
# Turn off all LEDs
GPIO.output(LedPins, GPIO.HIGH)
# Release resource
GPIO.cleanup()
# If run this script directly, do:
if __name__ == '__main__':
setup()
try:
main()
# When 'Ctrl+C' is pressed, the child program
# destroy() will be executed.
except KeyboardInterrupt:
destroy()