Introduction
Different from the last experiment, an ADC0832 and a Joystick PS2 are added here to make the measurement system more intelligent.
Components
– 1 * Raspberry Pi
– 1 * Breadboard
– 1 * Buzzer
– 1 * RGB LED
– 1 * DS18B20
– 1 * ADC0832
– 1 * Joystick PS2
– Several jumper wires
Experimental Principle
The principle is similar with that in the last experiment – only that you can adjust the lower and the upper limit value by the Joystick PS2 when programming.
Joystick PS2 has five operation directions: up, down, left, right and press-down. In this experiment, we will use left/right direction to control the upper limit value and up/down to control the lower limit value. If you press down the joystick, the system will log out.
Experimental Procedures
Step 1: Build the circuit

Raspberry Pi GPIO0 —————— ADC0832 pin CS
Raspberry Pi GPIO1 ——————-ADC0832 pin CLK
Raspberry Pi GPIO2 —————— ADC0832 pin DIO
Joystick PS2 pin x ——————— ADC0832 pin CH0
Joystick PS2 pin y ———————-ADC0832 pin CH1
Joystick PS2 pin z ———————- Raspberry Pi GPIO6
Raspberry Pi GPIO3 ——————— RGB LED pin R
Raspberry Pi GPIO4 ——————— RGB LED pin G
Raspberry Pi GPIO5 ——————— RGB LED pin B
Raspberry Pi GPIO8 ——————— Buzzer module pin S
Raspberry Pi GND———————— Buzzer module pin –
Step 2: Edit and save the code (see path/Rpi_SensorKit_Code/30_expand02/ tempMonitor.c)
Step 3: Compile
gcc tempMonitor.c -lwiringPi
Step 4: Run
./a.out
Now, push the knob left and right to set the upper limit value, and up and down to set the lower limit value. If the ambient temperature reaches the upper or lower limit value, the buzzer will beep at different frequencies.
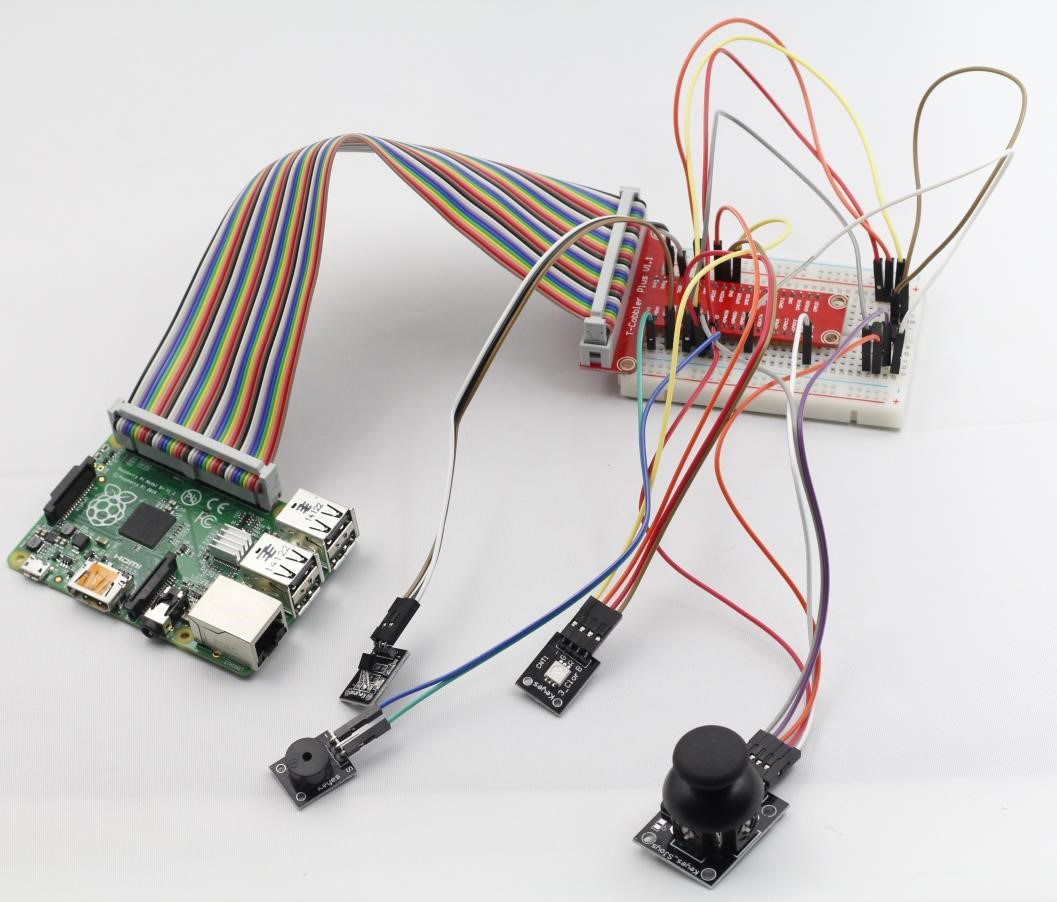
tempMonitor.c
#include <wiringPi.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <errno.h>
#include <stdlib.h>
#include <stdio.h>
#define LedRed 3
#define LedGreen 4
#define LedBlue 5
#define ADC_CS 0
#define ADC_CLK 1
#define ADC_DIO 2
#define JoyStick_Z 6
#define Beep 8
#define BUFSIZE 128
typedef unsigned char uchar;
typedef unsigned int uint;
static int sys_state = 1;
void beepInit(void)
{
pinMode(Beep, OUTPUT);
}
void beep_on(void)
{
digitalWrite(Beep, LOW);
}
void beep_off(void)
{
digitalWrite(Beep, HIGH);
}
void beepCtrl(int t)
{
beep_on();
delay(t);
beep_off();
delay(t);
}
float tempRead(void)
{
float temp;
int i, j;
int fd;
int ret;
char buf[BUFSIZE];
char tempBuf[5];
fd = open("/sys/bus/w1/devices/28-00000495db35/w1_slave", O_RDONLY);
if(-1 == fd){
perror("open device file error");
return 1;
}
while(1){
ret = read(fd, buf, BUFSIZE);
if(0 == ret){
break;
}
if(-1 == ret){
if(errno == EINTR){
continue;
}
perror("read()");
close(fd);
return 1;
}
}
for(i=0;i<sizeof(buf);i++){
if(buf[i] == 't'){
for(j=0;j<sizeof(tempBuf);j++){
tempBuf[j] = buf[i+2+j];
}
}
}
temp = (float)atoi(tempBuf) / 1000;
close(fd);
return temp;
}
void ledInit(void)
{
pinMode(LedRed, OUTPUT);
pinMode(LedGreen, OUTPUT);
pinMode(LedBlue, OUTPUT);
}
/* */
void ledCtrl(int n, int state)
{
digitalWrite(n, state);
}
uchar get_ADC_Result(uchar xyVal)
{
//10:CH0
//11:CH1
uchar i;
uchar dat1=0, dat2=0;
digitalWrite(ADC_CS, 0);
digitalWrite(ADC_CLK,0);
digitalWrite(ADC_DIO,1); delayMicroseconds(2);
digitalWrite(ADC_CLK,1); delayMicroseconds(2);
digitalWrite(ADC_CLK,0);
digitalWrite(ADC_DIO,1); delayMicroseconds(2); //CH0 10
digitalWrite(ADC_CLK,1); delayMicroseconds(2);
digitalWrite(ADC_CLK,0);
if(xyVal == 'x'){
digitalWrite(ADC_DIO,0); delayMicroseconds(2); //CH0 0
}
if(xyVal == 'y'){
digitalWrite(ADC_DIO,1); delayMicroseconds(2); //CH1 1
}
digitalWrite(ADC_CLK,1);
digitalWrite(ADC_DIO,1); delayMicroseconds(2);
digitalWrite(ADC_CLK,0);
digitalWrite(ADC_DIO,1); delayMicroseconds(2);
for(i=0;i<8;i++)
{
digitalWrite(ADC_CLK,1); delayMicroseconds(2);
digitalWrite(ADC_CLK,0); delayMicroseconds(2);
pinMode(ADC_DIO, INPUT);
dat1=dat1<<1 | digitalRead(ADC_DIO);
}
for(i=0;i<8;i++)
{
dat2 = dat2 | ((uchar)(digitalRead(ADC_DIO))<<i);
digitalWrite(ADC_CLK,1); delayMicroseconds(2);
digitalWrite(ADC_CLK,0); delayMicroseconds(2);
}
digitalWrite(ADC_CS,1);
pinMode(ADC_DIO, OUTPUT);
return(dat1==dat2) ? dat1 : 0;
}
void joyStick_z_ISR(void)
{
sys_state = 0;
printf("interrupt occur !\n");
}
uchar get_joyStick_state(void)
{
uchar tmp = 0;
uchar xVal = 0, yVal = 0, zVal = 0;
pinMode(ADC_DIO, OUTPUT);
xVal = get_ADC_Result('x');
if(xVal == 255){
tmp = 1;
}
if(xVal == 0){
tmp = 2;
}
yVal = get_ADC_Result('y');
if(yVal == 255){
tmp = 4;
}
if(yVal == 0){
tmp = 3;
}
return tmp;
}
int main(void)
{
int i;
uchar joyStick = 0;
float temp;
uchar low = 26, high = 30;
if(wiringPiSetup() == -1){
printf("setup wiringPi failed !");
return 1;
}
pullUpDnControl(JoyStick_Z, PUD_UP);
wiringPiISR(JoyStick_Z, INT_EDGE_FALLING, &joyStick_z_ISR);
pinMode(ADC_CS, OUTPUT);
pinMode(ADC_CLK, OUTPUT);
pinMode(ADC_DIO, OUTPUT);
ledInit();
beepInit();
printf("System is running...\n");
while(1){
flag:
joyStick = get_joyStick_state();
switch(joyStick){
case 1 : ++low; break;
case 2 : --low; break;
case 3 : --high; break;
case 4 : ++high; break;
default: break;
}
if(low >= high){
printf("Error, lower limit should be less than upper limit\n");
goto flag;
}
printf("The lower limit of temperature : %d\n", low);
printf("The upper limit of temperature : %d\n", high);
temp = tempRead();
printf("Current temperature : %0.3f\n", temp);
if(temp < low){
ledCtrl(LedBlue, HIGH);
ledCtrl(LedRed, LOW);
ledCtrl(LedGreen, LOW);
for(i = 0;i < 3; i++){
beepCtrl(400);
}
}
if(temp >= low && temp < high){
ledCtrl(LedBlue, LOW);
ledCtrl(LedRed, LOW);
ledCtrl(LedGreen, HIGH);
}
if(temp >= high){
ledCtrl(LedBlue, LOW);
ledCtrl(LedRed, HIGH);
ledCtrl(LedGreen, LOW);
for(i = 0;i < 3; i++){
beepCtrl(80);
}
}
if(sys_state == 0){
ledCtrl(LedRed, LOW);
ledCtrl(LedGreen, LOW);
ledCtrl(LedBlue, LOW);
beep_off();
printf("System will be off...\n");
break;
}
}
return 0;
}
Python Code
#!/usr/bin/env python
import RPi.GPIO as GPIO
import ADC0832
import time
import os
# Define RGB LED pin
LedRed = 15
LedGreen = 16
LedBlue = 18
# Define Joystick button pin
btn = 22
# Define Buzzer pin
Buzzer = 3
def beep(x):
GPIO.output(Buzzer, 0)
time.sleep(x)
GPIO.output(Buzzer, 1)
time.sleep(x)
def tempRead():
global ds18b20
address = '/sys/bus/w1/devices/' + ds18b20 + '/w1_slave'
tfile = open(address)
text = tfile.read()
tfile.close()
secondline = text.split("\n")[1]
temperaturedata = secondline.split(" ")[9]
temperature = float(temperaturedata[2:])
temperature = temperature / 1000
return temperature
def joystickbtn(ev=None):
print 'Interrupt occur!'
destroy()
def joystickState():
if ADC0832.getResult1() == 0:
return 1 #up
if ADC0832.getResult1() == 255:
return 2 #down
if ADC0832.getResult() == 0:
return 3 #left
if ADC0832.getResult() == 255:
return 4 #right
def map(x, in_min, in_max, out_min, out_max):
return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min
def ds18b20Init():
global ds18b20
for i in os.listdir('/sys/bus/w1/devices'):
if i != 'w1-bus-master1':
ds18b20 = i
def setup():
GPIO.setmode(GPIO.BOARD)
# Buzzer setup:
GPIO.setup(Buzzer, GPIO.OUT)
GPIO.output(Buzzer, 1)
# RGB setup:
GPIO.setup(LedRed, GPIO.OUT)
GPIO.setup(LedGreen, GPIO.OUT)
GPIO.setup(LedBlue, GPIO.OUT)
GPIO.output(LedRed, 1)
GPIO.output(LedGreen, 1)
GPIO.output(LedBlue, 1)
# DS18B20 setup:
ds18b20Init()
# Joystick setup:
GPIO.setup(btn, GPIO.IN)
GPIO.add_event_detect(btn, GPIO.FALLING, callback=joystickbtn, bouncetime=200)
# ADC0832 setup:
ADC0832.setup()
def loop():
low = 28
high = 32
print 'System is running...'
while True:
# Change low/high limit with Joystick
joystick = joystickState()
print joystick
if joystick == 1 and low < high - 1:
low += 1
if joystick == 2 and low > 0:
low -= 1
if joystick == 4 and high > low + 1:
high -= 1
if joystick == 3 and high < 125:
high += 1
print 'The lower limit of temperature:', low
print 'The upper limit of temperature:', high
# Read temperature from ds18B20
temp = tempRead()
print 'Current temperature:', temp
# Under/Over limit alarm:
if temp < low:
GPIO.output(LedBlue, 0);
GPIO.output(LedRed, 1);
GPIO.output(LedGreen, 1);
for i in range(0, 4):
beep(0.25)
if temp >= low and temp < high:
GPIO.output(LedBlue, 1);
GPIO.output(LedRed, 1);
GPIO.output(LedGreen, 0);
time.sleep(1)
if temp >= high:
GPIO.output(LedBlue, 1);
GPIO.output(LedRed, 0);
GPIO.output(LedGreen, 1);
for i in range(0, 8):
beep(0.125)
def destroy():
GPIO.output(LedRed, 1)
GPIO.output(LedGreen, 1)
GPIO.output(LedBlue, 1)
GPIO.output(Buzzer, 1)
GPIO.cleanup()
if __name__ == '__main__':
setup()
try:
loop()
except KeyboardInterrupt:
destroy()