Introduction
A vibration switch, also called spring switch or shock sensor, is an electronic switch which induces shock force and transfers the result to a circuit device thus triggering it to work. It contains the following parts: conductive vibration spring, switch body, trigger pin, and packaging agent.
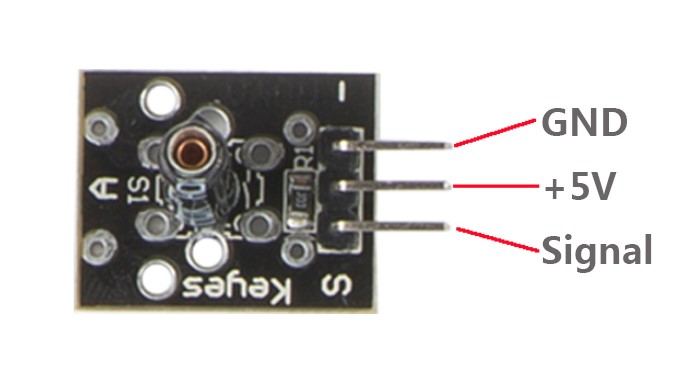
Components
– 1 * Raspberry Pi
– 1 * Breadboard
– 1 * Network cable (or USB wireless network adapter)
– 1 * Shock switch module
– 1 * Dual-color Common-Cathode LED module
– Jumper wires
Experimental Principle
The shock switch works like this: the conductive vibration spring and trigger pin are precisely placed in the switch and fixed by adhesive. Normally, the spring and the trigger pin are separated. Once the sensor detects shock, the spring will vibrate and contact with the trigger pin, thus conducting and generating trigger signals.
In this experiment, a dual-color LED module is used to indicate shock signals. When the shock switch inducts shock signals, the LED will light up.
Experimental Procedures
Step 1: Build the circuit
Shock switch connection: connect pin S of the Shock switch module to GPIO0 of the Raspberry Pi; GND to GND; pin + to 3.3V
Dual-color LED module connection: connect pin R of the dual-color LED module to GPIO1 of the Raspberry Pi; GND to GND
Step 2: Edit and save the code (see path/Rpi_SensorKit_code/06_shockSwitch/shockSwitch.c)
Step 3: Compile
gcc shockSwitch.c -lwiringPi
Step 4: Run
./a.out
Shake the switch and you will see the string “Detected shaking ! count = ?” printed on screen, and the LED will light up.
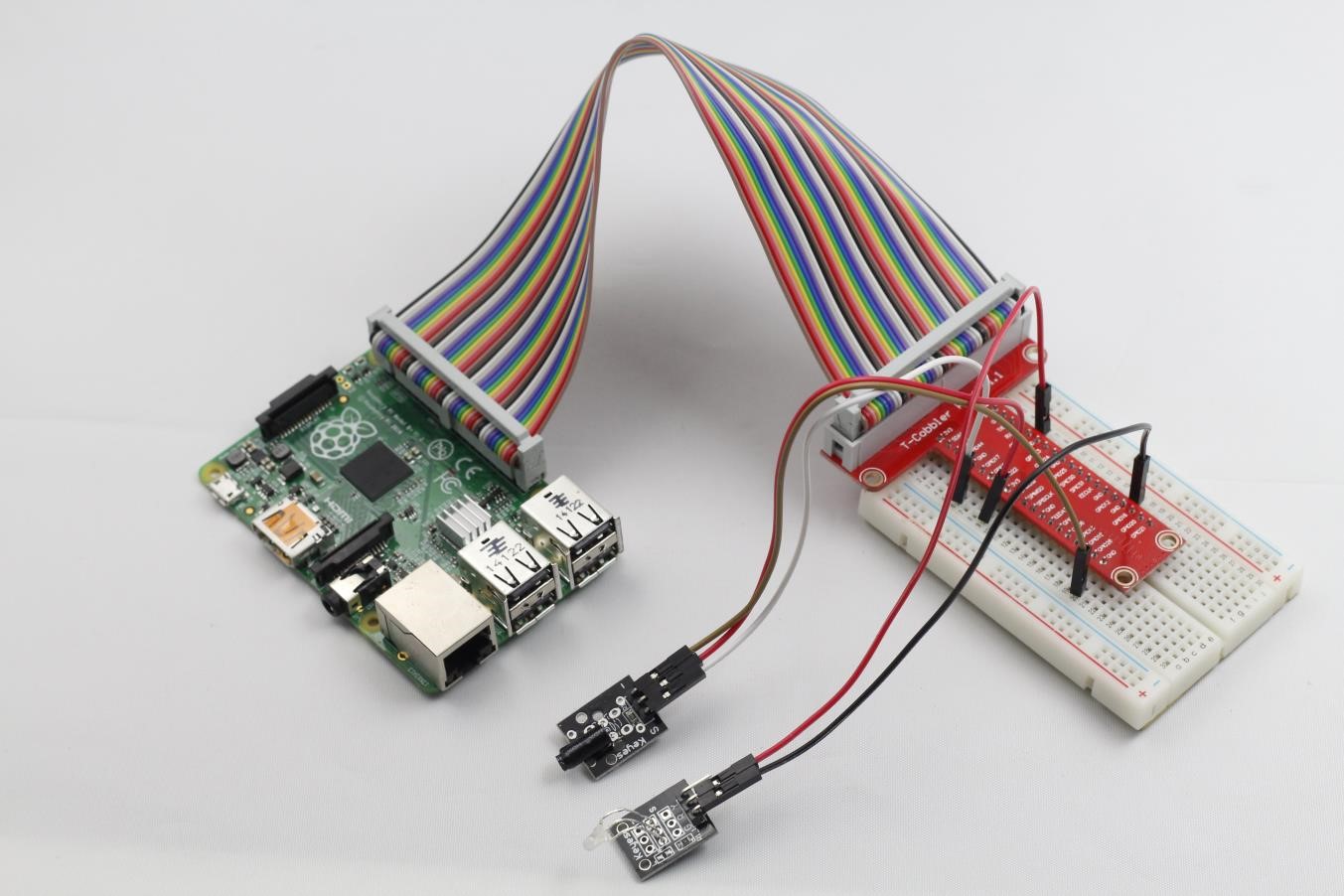
shockSwitch.c
#include <wiringPi.h>
#include <stdio.h>
#define ShockPin 0
#define LedPin 1
int main(void)
{
int count;
if(wiringPiSetup() == -1){ //when initialize wiring failed,print messageto screen
printf("setup wiringPi failed !");
return 1;
}
pinMode(ShockPin, INPUT);
pinMode(LedPin, OUTPUT);
while(1){
if(digitalRead(ShockPin) == LOW){
delay(10);
if(digitalRead(ShockPin) == LOW){
count++;
printf("Detected shaking ! count = %d\n", count);
digitalWrite(LedPin, !digitalRead(LedPin));
}
}
}
return 0;
}
Python Code
#!/usr/bin/env python
import RPi.GPIO as GPIO
ShockPin = 11
LedPin = 12
Led_status = 1
def setup():
GPIO.setmode(GPIO.BOARD) # Numbers GPIOs by physical location
GPIO.setup(LedPin, GPIO.OUT) # Set LedPin's mode is output
GPIO.setup(ShockPin, GPIO.IN, pull_up_down=GPIO.PUD_UP)
GPIO.output(LedPin, GPIO.HIGH) # Set LedPin high(+3.3V) to off led
def swLed(ev=None):
global Led_status
Led_status = not Led_status
GPIO.output(LedPin, Led_status) # switch led status(on-->off; off-->on)
print "led: " + ("on" if Led_status else "off")
def loop():
GPIO.add_event_detect(ShockPin, GPIO.FALLING, callback=swLed, bouncetime=200) # wait for falling
while True:
pass # Don't do anything
def destroy():
GPIO.output(LedPin, GPIO.LOW) # led off
GPIO.cleanup() # Release resource
if __name__ == '__main__': # Program start from here
setup()
try:
loop()
except KeyboardInterrupt: # When 'Ctrl+C' is pressed, the child program destroy() will be executed.
destroy()